radiosparks
Member
The ease at which to program the PICAXE is truly astounding. I thought a Morse code generator would be a “NO BRAINER” but was caught off guard. There are so many different ways to create this type of code.
Most programs need the user to map the ASCII to a binary code to create the data string. “That hurt my head.” I just wanted to enter a string of ASCII and let the MCU handle the mapping.
This was cobbled together from some interesting snippets of code found by scanning around the forum and READING all things of interest.
QUOTE: mrburnette: “It is by evolving concepts and existing code bases that code evolution occurs”
Discovering new ways to optimize my code to fit in a restricted microcontroller environment, like the PICAXE, is helping me to become a better programmer.
The RC network you see on the breadboard acts as a low pass filter to remove the harmonics and is just to soften the harsh tone from the piezo transducer.
There is more than enough memory to add more features. All the code doesn't fit in the post see part 2.
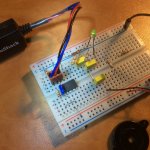
Most programs need the user to map the ASCII to a binary code to create the data string. “That hurt my head.” I just wanted to enter a string of ASCII and let the MCU handle the mapping.
This was cobbled together from some interesting snippets of code found by scanning around the forum and READING all things of interest.
QUOTE: mrburnette: “It is by evolving concepts and existing code bases that code evolution occurs”
Discovering new ways to optimize my code to fit in a restricted microcontroller environment, like the PICAXE, is helping me to become a better programmer.
The RC network you see on the breadboard acts as a low pass filter to remove the harmonics and is just to soften the harsh tone from the piezo transducer.
There is more than enough memory to add more features. All the code doesn't fit in the post see part 2.
Code:
#REM
-----------------------------------------------------------------------
PICAXE-08M2 Simplified Morse Code Generator - rhb.20230913
NOTE: Only alpha and numerals are used in this demonstration.
Other characters included are commented out.
This was to made to fit into an old 08M,
which was the original CW IDer for my 1W QRP rig.
-----------------------------------------------------------------------
NOTES: Physical Connections
pin C.1 LED output, or TR key out with transistor (2N3904) driver
pin C.2 SIDE-TONE output is a simple piezo speaker
-----------------------------------------------------------------------
Morse Code Alphanumeric Character Encoding
-----------------------------------------------------------------------
Each character to be generated is programmed as a number whose
binary equivalent then generates Morse code.
Test the MSB (Most Significant Bit) to represent dits and dahs.
dot = dit = 0
dash = dah = 1
One (1) bit on the end of the Morse Character to detect a stop
shifting left until the byte equals 128 ($80)
Example Letter Conversion:
k = -.- Morse pattern
k = 101 Replace dash and dots with binary 1 and 0
k = 1011 Add 1 bit to end
k = 10110000 Pad with 0 bits to make a 8 bit BYTE
k = %10110000 Defined data as binary value
k = $B0 Defined data as HEX value
k = 176 Defined data as Decimal value
-----------------------------------------------------------------------
Visual Table of Morse Code HEX Values
-----------------------------------------------------------------------
ASCII Shift-Left Code
Letter Morse Binary HEX
A .- 0110 0000 $60
B -... 1000 1000 $88
C -.-. 1010 1000 $A8
D -.. 1001 0000 $90
E . 0100 0000 $40
F ..-. 0010 1000 $28
G --. 1101 0000 $D0
H .... 0000 1000 $08
I .. 0010 0000 $20
J .--- 0111 1000 $78
K -.- 1011 0000 $B0
L .-.. 0100 1000 $48
M -- 1110 0000 $E0
N -. 1010 0000 $A0
O --- 1111 0000 $F0
P .--. 0110 1000 $68
Q --.- 1101 1000 $D8
R .-. 0101 0000 $50
S ... 0001 0000 $10
T - 1100 0000 $C0
U ..- 0011 0000 $30
V ...- 0001 1000 $18
W .-- 0111 0000 $70
X -..- 1001 1000 $98
Y -.-- 1011 1000 $B8
Z --.. 1100 1000 $C8
-----------------------------------------------------------------------
ASCII Shift-Left Code
Number Morse Binary HEX
0 ----- 1111 1100 $FC
1 .---- 0111 1100 $7C
2 ..--- 0011 1100 $3C
3 ...-- 0001 1100 $1C
4 ....- 0000 1100 $0C
5 ..... 0000 0100 $04
6 -.... 1000 0100 $84
7 --... 1100 0100 $C4
8 ---.. 1110 0100 $E4
9 ----. 1111 0100 $F4
-----------------------------------------------------------------------
Punctuation
-----------------------------------------------------------------------
e.g. (SK) Digraph are sent together without a character space
word space [space key] 1000 0000 $80
exclamation mark (KW)[!] -.-.-- 1010 1110 $AE
quotation mark ["] .-..-. 0100 1010 $4A
hashtag [#]
dollar sign (SX)[$] ...-..- 0001 0011 $13
percent [%]
ampersand (AS)[&] .-... 0100 0100 $44
apostrophe ['] .----. 0111 1010 $7A
parenthesis open (KN)[(] -.--. 1011 0100 $B4
parenthesis closed [)] -.--.- 1011 0110 $B6
asterisk [*]
plus (RN)[+] .-.-. 0101 0100 $54
comma [,] --..-- 1100 1110 $CE
hyphen minus [-] -....- 1000 0110 $86
full stop (period) [.] .-.-.- 0101 0110 $56
forward slash (DN)[/] -..-. 1001 0100 $94
colon [:] ---... 1110 0010 $E2
semicolon [;] -.-.-. 1010 1010 $AA
less than [<]
equal sign (BT)[=] -...- 1000 1100 $8C
greater than [>]
question mark [?] ..--.. 0011 0010 $32
at-sign (commat) (AC)[@] .--.-. 0110 1010 $6A
underscore [_] ..--.- 0011 0110 $36
-----------------------------------------------------------------------
Procedural
-----------------------------------------------------------------------
Start of work (CT) -.-.- 1010 1010 $AA
End of work (SK) ...-.- 0001 0110 $16
Invitation to transmit (K) -.- 1011 0000 $B0
Invitation for a particular
station to transmit
(KN) -.--. 1011 0100 $B4
End of message (AR) .-.-. 0101 0100 $54
Wait (AS) .-... 0100 0100 $44
Understood (SN) ...-. 0001 0100 $14
Error (8 dits) (HH) ........ 1111 1111 $FF
-----------------------------------------------------------------------
Traditional Timing
-----------------------------------------------------------------------
1 dit = 1 dit
1 dah = 3 dits
letter space = 3 dits
word spacing = 7 dits
-----------------------------------------------------------------------
Farnsworth Timing
-----------------------------------------------------------------------
letter space = 9 dits
word spacing = 21 dits
-----------------------------------------------------------------------
#ENDREM
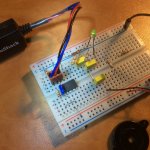