I recieved some RGB LEDs recently, but my 18M2 only has 2 pins capable of PWM. I wrote this code to cycle through the colors. Is there a better way? 
The circuit consists of the Picaxe, four transistors and the LED plus a resistor.
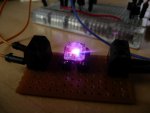
This picture shows the transistor configuration (the 1s are the position of the pin in the pinsB)
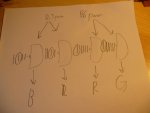
Here is a video of the result:
Code:
let dirsB =%11111111
main:
let pinsB =%00100010
pwmout B.6,150,0
pwmout B.3,150,255
'red out green in
for b0 = 255 to 0 STEP -1
let b1 = 255 - b0
pwmduty B.6,b1
pwmduty B.3,b0
next b0
let pinsB =%00100100
'green out blue in
pwmout B.3,150,0
for b0 = 255 to 0 STEP -1
let b1 = 255 - b0
pwmduty B.6,b0
pwmduty B.3,b1
next b0
'blue out, red in'
let pinsB =%00010100
pwmout B.3,150,0
pwmout B.6,150,0
for b0 = 255 to 0 STEP -1
let b1 = 255 - b0
pwmduty B.3,b0
pwmduty B.6,b1
next b0
goto main
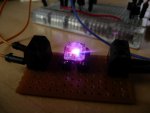
This picture shows the transistor configuration (the 1s are the position of the pin in the pinsB)
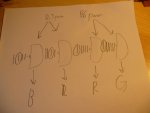
Here is a video of the result: