Hairy Animal
Member
Hi, I'm trying to make a (relatively) simple dual pump controller which essentially has two water sensors and two pumps each pumping water from different areas.
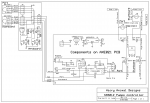
I want each pump to start independently only after its sensor has gone high (sensed water) for at least a second, and continue pumping until after the sensor has gone low again for a delayed period set by a toggle switch (1, 3, or 6 minutes). I'm using a 08M2 because I have lots of them and associated PCBs, and there are just enough pins, BUT, I do need to use the C.5 input which of course is normally used as part of the serial programming interface and therefore use the DISCONNECT command after a delay in the initialisation.
It struck me that it might be a good idea to use parallel tasks to handle the two pumps (effectively) simultaneously, but then discovered that I need to start the program with the first task; i.e. I can't do any of the initialisation tasks first.
So I made task #0 the initialisation and introduced two further tasks for the two pump controllers, only to realise that all three tasks would start simultaneously, not at all what I'd intended. Code below is an outline of what I'd intended, but is unlikely to work.
I'd be most grateful for suggestions as to a better way to achieve this more elegantly, interrupts maybe?
TIA.
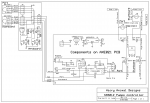
I want each pump to start independently only after its sensor has gone high (sensed water) for at least a second, and continue pumping until after the sensor has gone low again for a delayed period set by a toggle switch (1, 3, or 6 minutes). I'm using a 08M2 because I have lots of them and associated PCBs, and there are just enough pins, BUT, I do need to use the C.5 input which of course is normally used as part of the serial programming interface and therefore use the DISCONNECT command after a delay in the initialisation.
It struck me that it might be a good idea to use parallel tasks to handle the two pumps (effectively) simultaneously, but then discovered that I need to start the program with the first task; i.e. I can't do any of the initialisation tasks first.
So I made task #0 the initialisation and introduced two further tasks for the two pump controllers, only to realise that all three tasks would start simultaneously, not at all what I'd intended. Code below is an outline of what I'd intended, but is unlikely to work.
I'd be most grateful for suggestions as to a better way to achieve this more elegantly, interrupts maybe?
TIA.
Code:
START0: ; Task #0 - initialisation.
; Task #1 - port pump control.
; Task #2 - starboard pump control.
;
GOSUB demo_test
PAUSE 5000 ; Five second delay to allow for programming
; to happen at power up before the DISCONNECT command.
;
DISCONNECT ; This allows the C.5 input to be used without
; interference from the firmware looking for
; programming input.
; ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
START1: ; Task #1 - port pump control.
IF Port_Sens = 1 THEN
GOSUB P_on ELSE
GOSUB P_off
ENDIF
GOTO START1
; ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
START2: ; Task #2 - starboard pump control.
;
;
IF Stbd_Sens = 1 THEN
GOSUB S_on ELSE
GOSUB S_off
ENDIF
GOTO START2
; ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
END